blob: 015d0d8edbd3a2c2bbe92f049256bb09283e50aa (
plain)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
|
# KAU :kpref-activity
KAU supports Preferences that are created without xmls and through AppCompat.
The items are backed by a [FastAdapter](https://github.com/mikepenz/FastAdapter) and support [iicons](https://github.com/mikepenz/Android-Iconics)
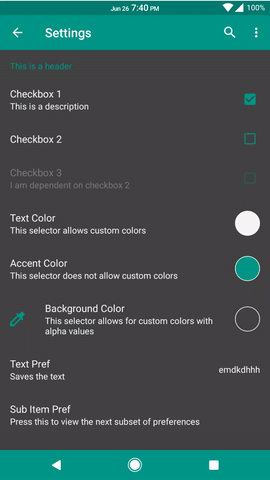
The easiest way to create the settings is to extend `KPrefActivity`.
We will then override `onCreateKPrefs` to generate our adapter builder.
The adapter builder can easily add items using defined functions.
Each item added extends one or more contracts to configure it.
The contracts are as follows:
Contract | Mandatory | Optional | Description
:--- | :--- | :--- | :---
`CoreAttributeContract` | `NA` | `textColor` `accentColor` | Defines stylings that are added in every item
`CoreContract` | `titleRes` | `descRes` `iicon` | Implemented by every item
`BaseContract` | `getter` `setter` | `enabler` `onClick` `onDisabledClick` | Implemented by every preference item
`KPrefColorContract` | `NA` | `showPreview` | Additional configurations for the color picker
`KPrefTextContract` | `NA` | `textGetter` | Additional configurations for the text item
`KPrefSubItemsContract` | `itemBuilder` | `NA` | Contains a new list for the adapter to load when clicked
The kpref items are as followed:
Item | Implements | Description
:--- | :--- | :---
`checkbox` | `CoreContract` `BaseContract` | Checkbox item; by default, clicking it will toggle the checkbox and the kpref
`colorPicker` | `CoreContract` `BaseContract` `KPrefColorContract` | Color picker item; by default, clicking it will open a dialog which will change the color (int)
`header` | `CoreContract` | Header; just a title that isn't clickable
`text` | `CoreContract` `BaseContract` `KPrefTextContract` | Text item; displays the kpref as a String on the right; does not have click implementation by default
`plainText` | `BaseContract` | Plain text item; like `text` but does not deal with any preferences directly, so it doesn't need a getter or setter
This can be used to display text or deal with preference that are completely handed within the click event (eg a dialog).
`subItems` | `CoreContract` `KPrefSubItemsContract` | Sub items; contains a new page for the activity to load when clicked
An example of the adapter builder:
```kotlin
override fun onCreateKPrefs(savedInstanceState: android.os.Bundle?): KPrefAdapterBuilder.() -> Unit = {
header(R.string.header)
/**
* This is how the setup looks like with all the proper tags
*/
checkbox(title = R.string.checkbox_1, getter = { KPrefSample.check1 }, setter = { KPrefSample.check1 = it },
builder = {
descRes = R.string.desc
})
/**
* This is how it looks like without the tags
*/
checkbox(R.string.checkbox_3, { KPrefSample.check3 }, { KPrefSample.check3 = it }) {
descRes = R.string.desc_dependent
enabler = { KPrefSample.check2 }
onDisabledClick = {
itemView, _, _ ->
itemView.context.toast("I am still disabled")
true
}
}
}
```
On top of per item configurations, `KPrefActivity` has some core attributes that you can define on creation.
It is done through the abstract function:
```kotlin
override fun kPrefCoreAttributes(): CoreAttributeContract.() -> Unit = {
textColor = { Prefs.textColor } // text color getter; refreshes automatically on reload
accentColor = { Prefs.accentColor } // accent color getter
// background color does not exist as it is done through the ripple canvas
}
```
|